Synonyms and Antonyms in Python
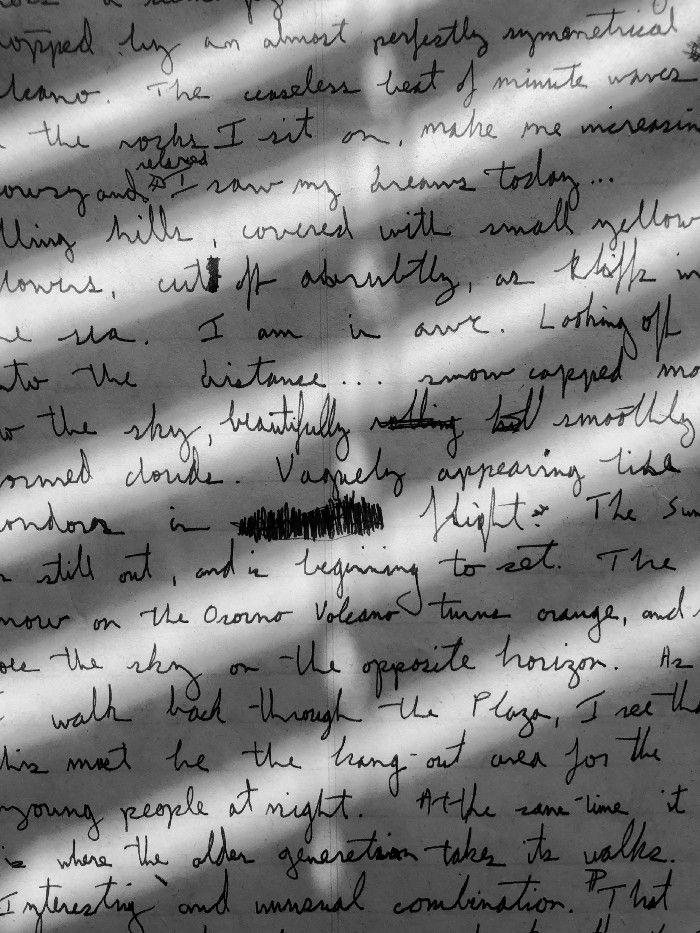
Language analysis can be carried out in many ways. In this blog, we will see how to extract Synonyms and Antonyms from the text using Natural Language Processing(NLTK) WordNet library.
Source: Vincent Russo
The WordNet is a part of Python’s Natural Language Toolkit. It is a large collection of words and vocabulary from the English language that are related to each other and are grouped in some way. A collection of similar words is called lemmas. Also, It’s a combination of dictionary and thesaurus. It is used for automatic text analysis and artificial intelligence applications. It supports many other languages in its collection. Please check for more information about WordNet here
Nouns, verbs, adjectives, and adverbs are grouped into sets of cognitive synonyms (synsets), each expressing a distinct concept. Synsets are interlinked by means of conceptual-semantic and lexical relations. Let’s see some examples
To find the meaning of the word
Code
Import NLTK library and install Wordnet
import nltk nltk.download('wordnet')
In this example, we will see how wordnet returns meaning and other details of the word. Let’s go ahead and look up the word “travel”
Sometimes, if some examples are available, it may also provide that.#Checking the word "Teaching"syn = wordnet.synsets(“teaching”) syn
Output[Synset('teaching.n.01'), Synset('teaching.n.02'), Synset('education.n.01'), Synset('teach.v.01'), Synset('teach.v.02')]
We can see that “TEACHING” has five meanings. Let’s find the first sense to get a better understanding of the kind of information each synset contains. We can do this by indexing the first element at its name. n, v represents Parts of speech tagging.
Code# Printing the Synonym, meaning and example of "teaching" for the first two indexes#First Indexprint(‘Word and Type : ‘ + syn[0].name()) print(‘Synonym of teaching is: ‘ + syn[0].lemmas()[0].name()) print(‘The meaning of the teaching: ‘ + syn[0].definition()) print(‘Example of teaching : ‘ + str(syn[0].examples()))#Second Indexprint(‘Word and Type : ‘ + syn[1].name()) print(‘Synonym of teaching is: ‘ + syn[1].lemmas()[0].name()) print(‘The meaning of the teaching : ‘ + syn[1].definition()) print(‘Example of teaching : ‘ + str(syn[1].examples()))
Output# Output for first indexWord and Type : teaching.n.01 Synonym of Teaching is: teaching The meaning of the Teaching: the profession of a teacher Example of Teaching : ['he prepared for teaching while still in college', 'pedagogy is recognized as an important profession']# Output for second indexWord and Type : teaching.n.02 Synonym of Teaching is: teaching The meaning of the Teaching : a doctrine that is taught Example of Teaching : ['the teachings of religion', 'he believed all the Christian precepts']
Synonyms
We can use lemmas()
the function of the synset. It returns synonyms as well as antonyms of that particular synset.
Code#Checking synonym for the word "travel"from nltk.corpus import wordnet#Creating a list synonyms = []for syn in wordnet.synsets("travel"): for lm in syn.lemmas(): synonyms.append(lm.name())#adding into synonyms print (set(synonyms))
Output{'trip', 'locomote', 'jaunt', 'change_of_location', 'go', 'traveling', 'travelling', 'locomotion', 'travel', 'move', 'journey', 'move_around'}
We can see the synonyms for the word ‘travel’ from the above output.
Antonyms
Code#Checking antonym for the word "increase"from nltk.corpus import wordnet antonyms = []for syn in wordnet.synsets("increase"): for lm in syn.lemmas(): if lm.antonyms(): antonyms.append(lm.antonyms()[0].name()) #adding into antonymsprint(set(antonyms))
Output{'decrement', 'decrease'}
WordNet has other feature called word similarity. It helps us check the similarity between two words that I didn’t cover in this blog.
Thanks for reading. Keep learning and stay tuned for more!